DETERMINE SALESFORCE RECORD TYPE BASED ON PICKLIST VALUE
For security reasons, the Lightning Component framework places restrictions on making API calls from JavaScript code. Using a named credential for specific API calls allows to carefully and selectively bypass this security restriction.
Business Case:
Client wants to auto-populate record type on standard case creation page on the basis of selected picklist value by using lightning component.
Solution:
As we can’t make API calls directly from Lightning Components so we ended up with learning it’s quite a complex process. We had to create three independent things to get this done:
- A connected App
- An Auth. Provider
- A Named Credential
Step 1: Create Connected App like this:
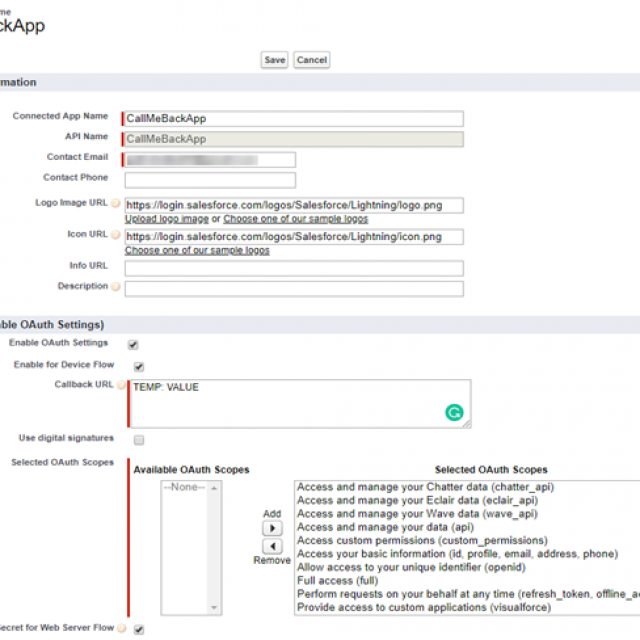
Note: Enter TEMP:VALUE for the Callback URL, we’ll change after auth. Provider creation.
Step 2: Create Auth. Provider as per given below:
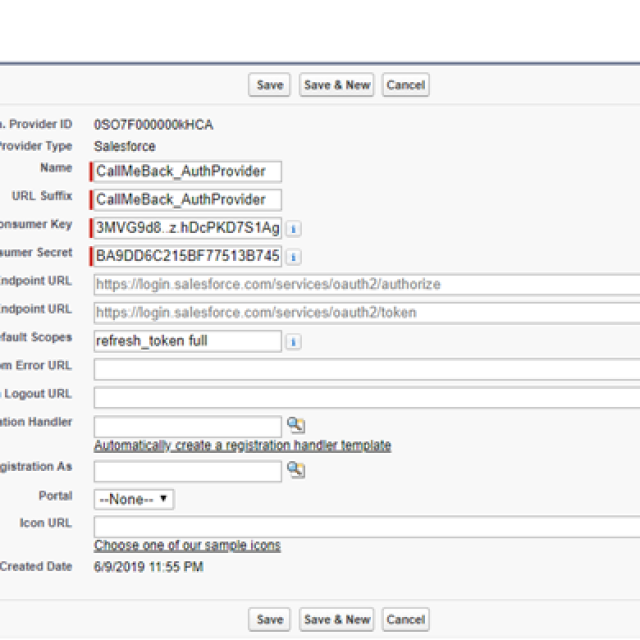
Copy-paste the consumer key and customer secret from the connected tab that we created above. For the Default Scopes type “refresh_token full”. And click save. You get to this screen:
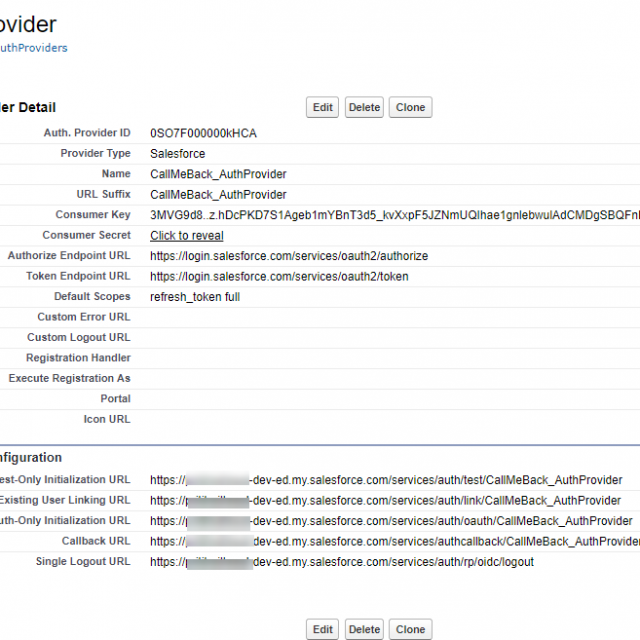
Copy the callback URL and paste this value in connected app Callback URL.
Step 3: Create Named Credentials:
Enter the URL for the ORG but make sure it’s ***. my.salesforce.com then replace .my.salesforce.com as per given below.
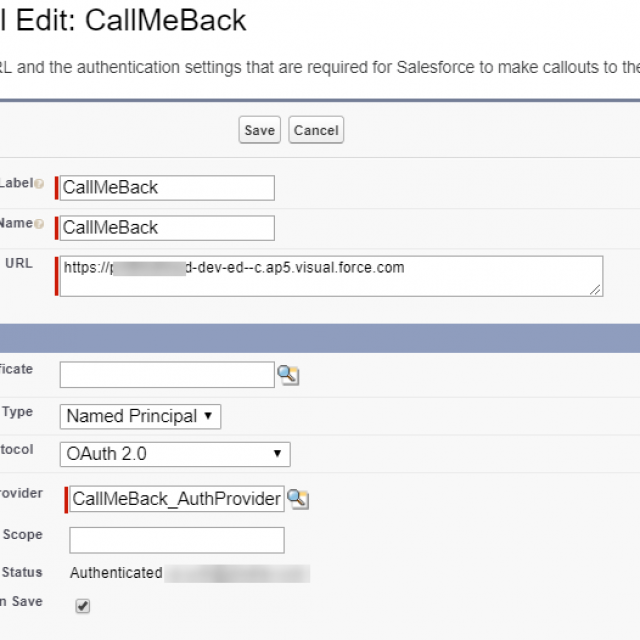
Step 4: Method to get Recordtype by picklist value.
Suppose picklist name is Category below method received parameter as category label and return record typeid.
public static String getRecordTypeIdForCategory(String categoryLabel) {
// results will contain HTML encoded characters, like &
categoryLabel = categoryLabel.escapeHtml4();
String recordTypeId = null;
Boolean foundMatch = false;
List<Id> recordTypeIds = new List<Id>();
//query all recordtypes of case object.
for(RecordType r : [SELECT Id, DeveloperName FROM RecordType where sObjectType=’Case’ AND IsActive = True]){
recordTypeIds.add(r.Id);
}
String host = System.Url.getSalesforceBaseURL().toExternalForm();
Map<String, List<String>> mapCategoriesByRecordType = new Map<String, List<String>>();
// create a map of Categories assigned to RecordTypes
for(String rId: recordTypeIds) {
Http http = new Http();
HttpRequest request = new HttpRequest();
Http h = new Http();
String url = ‘callout:CallMeBack/services/data/v42.0/ui-api/object-info/Case/picklist-values/’ + rId + ‘/Category__c’;
request.setEndpoint(url);
request.setMethod(‘GET’);
request.setHeader(‘Authorization’, ‘Bearer ‘+ UserInfo.getSessionId());
HttpResponse response;
response = http.send(request);
if(response.getStatus() == ‘OK’)
{
Map<String, Object> meta = (Map<String, Object>) JSON.deserializeUntyped(response.getBody());
List<String> categoriesList = new List<String>();
if(meta.containsKey(‘values’)){
for(Object o: (List<Object> )meta.get(‘values’)){
Map<String, object > temp = (Map<String, object>)o;
categoriesList.add((String)temp.get(‘label’));
}
}
mapCategoriesByRecordType.put(rid, categoriesList);
}
}
for (Id key : mapCategoriesByRecordType.keySet()) {
if(foundMatch == false) {
List<String> typeCategories = mapCategoriesByRecordType.get(key);
if(containsIgnoreCase(typeCategories, categoryLabel)) {
foundMatch = true;
recordTypeId = key;
}
}
}
return recordTypeId;
}
Output:
After selecting category in below component this will redirect to standard case creation page with auto-populating record type.
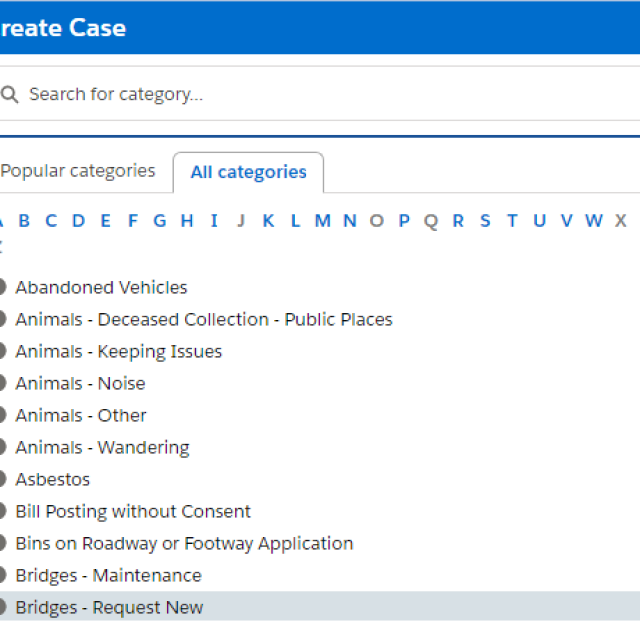